Hi everyone! It’s been a while since my last post, but I’m back with new curiosity and a fresh topic to explore. This time, I want to dive into Python, a programming language that has caught my interest.
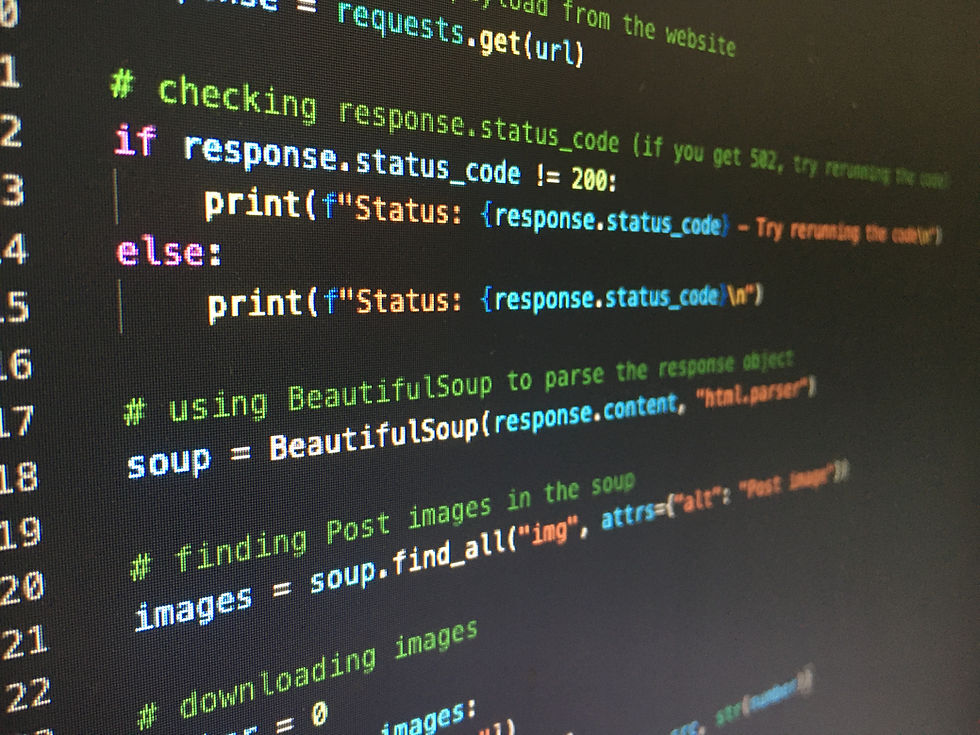
Why Python?
Python is just one of many programming languages, alongside Java, MATLAB, C, C++, C#, and many others. However, in this blog, I will focus only on Python—because it's open-source, versatile, and works across different operating systems, including MacOS, Windows, and Linux.
What is Python Used For?
Python is widely used to solve complex problems that would take too much time or effort to handle manually. Imagine you need to rename 100 folders on your PC—doing it manually would be exhausting, right? With Python, you can automate this task in seconds!
But before diving deeper, let's start with the basics of Python, beginning with data types.
Data Types in Python
Data types define what kind of data a variable can store. Python has several built-in data types:
Text Type: str → Used for processing text (e.g., "Hello, World!")
Numeric Types: int, float, complex → Used for numbers
Sequence Types: list, tuple, range → Used for ordered collections
Mapping Type: dict → Used for key-value pairs
Set Types: set, frozenset → Used for unordered collections of unique values
Boolean Type: bool→ Represents True or False
Binary Types: bytes, bytearray, memoryview → Used for handling binary data
None Type: NoneType → Represents the absence of a value
Understanding data types is crucial because it determines how Python processes and manipulates data.
Modules in Python
A module is simply a Python file (.py) that contains functions, variables, or classes that we can reuse. It helps us organize our code better.
Example: Creating a Module
Let's create a module called `calculator.py`:
```python
def add(a, b):
return a + b
def subtract(a, b):
return a - b
```
Now, if we want to use this module in another script, we import it:
```python
import calculator
result = calculator.add(5, 3)
print(result) # Output: 8
```
Modules make coding more efficient by allowing us to reuse functions instead of rewriting them.
What is a Library in Python?
A library is a collection of modules that provide various functionalities. Some libraries are built-in, while others need to be installed separately.
Popular Python Libraries:
TensorFlow – Machine learning & deep learning (developed by Google)
Matplotlib– Data visualization (static, animated, interactive)
Pandas– Data manipulation & analysis (great for handling tables)
NumPy– Working with arrays & mathematical operations
SciPy – Advanced scientific computing & engineering
Scikit-Learn – Machine learning models & data analysis
PyTorch – Deep learning with an intuitive interface
Libraries help simplify coding by providing pre-written functions so we don’t have to build everything from scratch!
What's Next?
Python can be used for many things, including web development, data engineering, data science, machine learning, and deep learning.
In my next blog post, I’ll introduce Machine Learning and Deep Learning—how they work and how Python plays a role in them.
Let’s learn together, and feel free to leave comments or suggestions if anything needs improvement. I'm always open to discussions! 😊
See you in the next blog! ✨
Written by : Indah Mustika Dewi
References:
Commentaires